编写Redis代码时候遇到一个需求,需要将缓存数据分批插入不同的数据库中。故收集了代码,并进行了测试!特别说明:Jedis必须使用JedisPool,否则会遇到线程安全问题!
环境准备:相关Maven的依赖
<!-- springboot中的redis依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- jedis依赖-->
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.6.0</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.6.2</version> <!--注意此版本过低将无法使用-->
</dependency>
说明:我们是通过Jedis 来进行不同的数据库插入
相关代码
初始化Jedis连接池 配置文件
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
@Configuration
public class JedisConfig {
@Bean
public JedisPool getJedisPool() {
JedisPoolConfig config = new JedisPoolConfig();
// 设置最小空闲对象连接数
config.setMinIdle(0);
// 最大空闲连接数
config.setMaxIdle(10);
// 最大连接数量 此配置需要参考Redis的配置 maxclients 一般来说maxclients是10000
config.setMaxTotal(5000);
// 设置最大阻塞时间,单位是毫秒
config.setMaxWaitMillis(1000 * 60);
JedisPool pool = new JedisPool(config, Host, Port, 超时时间(毫秒,默认是2000),Redis数据库密码);
return pool;
}
}
JedisUtil 如果需要其他功能需要自己封装
import com.google.gson.Gson;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
/**
* JedisUtil 主要功能是 redis跨库插值
*/
@Component
public class JedisUtil {
@Autowired
private JedisPool jedisPool;
/** 不设置过期时长 */
public final static int NOT_EXPIRE = -1;
/** 过期时长*/
public final static int EXRP_WEEK = 7 * 60 * 60 * 24;
private final static Gson gson = new Gson();
/**
* 插入值-对象,指定数据库 指定过期时间
*
* @param key 键
* @param value 值
* @param dbIndex 数据库索引 范围 0-15 默认0
* @param expire 过期时间 单位:秒
*/
public void set(String key, Object value, Integer dbIndex, int expire) {
Jedis jedis = getJedis(dbIndex);
jedis.set(key, toJson(value));
if (expire != NOT_EXPIRE) {
jedis.expire(key, expire);
}
}
/**
* 插入值-对象,指定数据库索引
*/
public void set(String key, Object value, Integer dbIndex) {
set(key, value, dbIndex, NOT_EXPIRE);
}
/**
* 获取值-对象,指定数据库索引,并设置过期时间
* @return
*/
public <T> T get(String key, Class<T> clazz, Integer dbIndex, int expire) {
Jedis jedis = getJedis(dbIndex);
try {
String value = jedis.get(key);
if (expire != NOT_EXPIRE) {
jedis.expire(key, expire);
}
return value == null ? null : fromJson(value, clazz);
} finally {
jedis.close();
}
}
/**
* 取值-对象 指定数据库索引
*/
public <T> T get(String key, Class<T> clazz, Integer dbIndex) {
return get(key, clazz, dbIndex, NOT_EXPIRE);
}
/**
* 取值-字符串,指定数据库索引,设置过期时间
*/
public String get(String key, Integer dbIndex, int expire) {
Jedis jedis = getJedis(dbIndex);
try {
String value = jedis.get(key);
if (expire != NOT_EXPIRE) {
jedis.expire(key, expire);
}
return value;
} finally {
jedis.close();
}
}
/**
* 取值-字符串,指定数据库索引
*/
public String get(String key, Integer dbIndex) {
return get(key, dbIndex, NOT_EXPIRE);
}
/**
* 删除,指定索引
*/
public void delete(String key, Integer dbIndex) {
Jedis jedis = getJedis(dbIndex);
try {
jedis.del(key);
} finally {
jedis.close();
}
}
/**
* 获取jedis对象,并指定dbIndex
*
* @param dbIndex
*/
private Jedis getJedis(Integer dbIndex) {
Jedis jedis = jedisPool.getResource();
if (dbIndex == null || dbIndex > 15 || dbIndex < 0) {
dbIndex = 0;
}
jedis.select(dbIndex);
return jedis;
}
/**
* Object转成JSON数据
*/
private String toJson(Object object) {
if (object instanceof Integer || object instanceof Long || object instanceof Float || object instanceof Double
|| object instanceof Boolean || object instanceof String) {
return String.valueOf(object);
}
return gson.toJson(object);
}
/**
* JSON数据,转成Object
*/
private <T> T fromJson(String json, Class<T> clazz) {
return gson.fromJson(json, clazz);
}
}
测试代码 jedisUtil.set(“Key“,”Value“,DB_Number,TTL);
import com.xunliao.RunApplication;
import com.xunliao.utils.JedisUtil;
import org.junit.Test;
import org.junit.runner.RunWith;
import com.xunliao.RunApplication;
import com.xunliao.utils.JedisUtil;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
@RunWith(SpringRunner.class)
@SpringBootTest(classes = {RunApplication.class})
public class JedisTest {
@Autowired
private JedisUtil jedisUtil;
@Test
public void contextLoads() {
//参数以此是 Key Value 数据库序号 超时时间(秒)
for (int i = 0; i < 99; i++) {
jedisUtil.set("key"+i,"value"+i,1,60);
jedisUtil.set("key"+i,"value"+i,2,120);
}
}
}
Redis 数据库结果
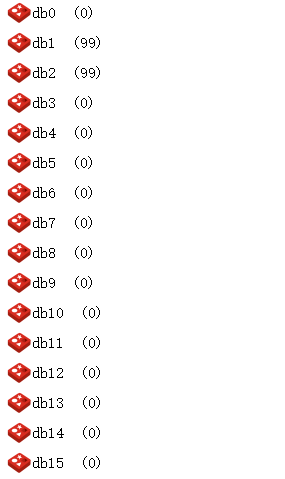
完成
特殊说明: 上述文章均是作者实际操作后产出。烦请各位,请勿直接盗用!转载记得标注原文链接:www.zanglikun.com
第三方平台不会及时更新本文最新内容。如果发现本文资料不全,可访问本人的Java博客搜索:标题关键字。以获取全部资料 ❤
第三方平台不会及时更新本文最新内容。如果发现本文资料不全,可访问本人的Java博客搜索:标题关键字。以获取全部资料 ❤
评论(0)